EFQRCode is a lightweight, pure-Swift library for generating stylized QRCode images with watermark or icon, and for recognizing QRCode from images, inspired by qrcode. Based on CoreGraphics
, CoreImage
, and ImageIO
, EFQRCode provides you a better way to handle QRCode in your app, no matter if it is on iOS, macOS, watchOS, and/or tvOS. You can integrate EFQRCode through CocoaPods, Carthage, and/or Swift Package Manager.
Awesome-qr.js is inspired by EFQRCode by EyreFree. EFQRCode is a tool to generate QRCode image or recognize QRCode from image, in Swift. If your application is in need of generating pretty QR codes in Swift, take a look at EFQRCode. AwesomeQRCode: Designed for Android.
Examples
Travis CI enables your team to test and ship your apps with confidence. Easily sync your projects with Travis CI and you'll be testing your code in minutes. Licenses for Open Source Development. JetBrains supports non-commercial open source projects by providing core project contributors with a set of best-in-class developer tools free of charge. EFQRCode, the app I want to share with you today, can help you make customized QR code with ease. Read More → You can also make individualized QR code without asking a designer: EFQRCode.
Demo Projects
App Store
You can click the App Store
button below to download demo, support iOS, tvOS and watchOS:
You can also click the Mac App Store
button below to download demo for macOS:
Compile Demo Manually
To run the example project manually, clone the repo, demos are in the ‘Examples’ folder, remember run command sh Startup.sh
in terminal to get all dependencies first, then open EFQRCode.xcworkspace
with Xcode and select the target you want, run.
Or you can run the following command in terminal:
Requirements
Version | Needs |
---|---|
1.x | Xcode 8.0+ Swift 3.0+ iOS 8.0+ / macOS 10.11+ / tvOS 9.0+ |
4.x | Xcode 9.0+ Swift 4.0+ iOS 8.0+ / macOS 10.11+ / tvOS 9.0+ / watchOS 2.0+ |
5.x | Xcode 11.1+ Swift 5.0+ iOS 8.0+ / macOS 10.11+ / tvOS 9.0+ / watchOS 2.0+ |
6.x | Xcode 12.0+ Swift 5.1+ iOS 9.0+ / macOS 10.10+ / tvOS 9.0+ / watchOS 2.0+ |
Installation
CocoaPods
EFQRCode is available through CocoaPods. To installit, simply add the following line to your Podfile:
Then, run the following command:
Carthage
IMPORTANT: this workaround is necessary for Carthage to somewhat work in Xcode 12.
Carthage is a decentralized dependency manager that builds your dependencies and provides you with binary frameworks.
You can install Carthage with Homebrew using the following command:
To integrate EFQRCode into your Xcode project using Carthage, specify it in your Cartfile
:
Run carthage update
to build the framework and drag the built EFQRCode.framework
into your Xcode project.
Swift Package Manager
The Swift Package Manager is a tool for automating the distribution of Swift code and is integrated into the Swift compiler.
Once you have your Swift package set up, adding EFQRCode as a dependency is as easy as adding it to the dependencies
value of your Package.swift
.
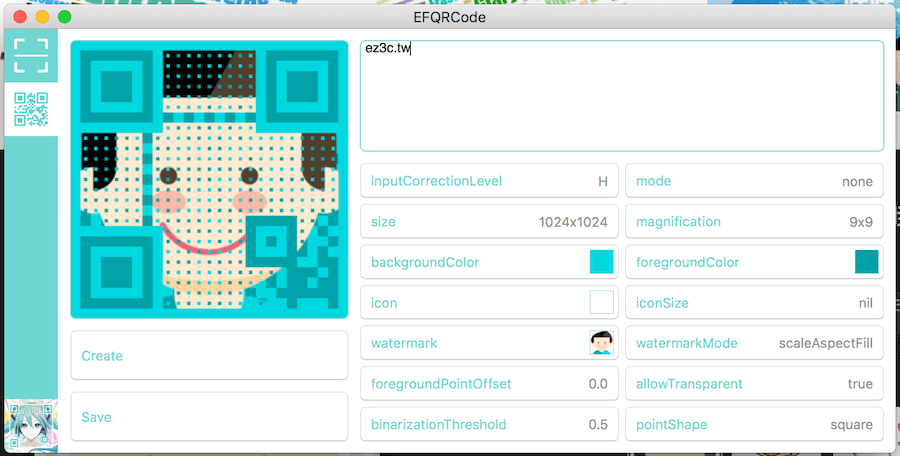
Quick Start
1. Import EFQRCode
Import EFQRCode module where you want to use it:

2. Recognition
A String Array is returned as there might be several QR Codes in a single CGImage
:
3. Generation
Create QR Code image, basic usage:
Parameter | Description |
---|---|
content | REQUIRED, content of QR Code |
size | Width and height of image |
backgroundColor | Background color of QRCode |
foregroundColor | Foreground color of QRCode |
watermark | Background image of QRCode |
Result:
4. Generation from GIF
Use EFQRCode.generateGIF
to create GIF QRCode.
Parameter | Description |
---|---|
generator | REQUIRED, an EFQRCodeGenerator instance with other settings |
data | REQUIRED, encoded input GIF |
delay | Output QRCode GIF delay, emitted means no change |
loopCount | Times looped in GIF, emitted means no change |
You can get more information from the demo, result will like this:
5. Next
Learn more from User Guide.
Recommendations
- Please select a high contrast foreground and background color combinations;
- To improve the definition of QRCode images, increase
size
, or scale up usingmagnification
(instead); - Magnification too high/size too large/contents too long may cause failure;
- It is recommended to test the QRCode image before put it into use;
- You can contact me if there is any problem, both
Issue
andPull request
are welcome.
PS of PS: I wish you can click the Star
button if this tool is useful for you, thanks, QAQ…
Other Platforms/Languages
Platforms/Languages | Link |
---|---|
Objective-C | https://github.com/z624821876/YSQRCode |
Java | https://github.com/SumiMakito/AwesomeQRCode |
JavaScript | https://github.com/SumiMakito/Awesome-qr.js |
Kotlin | https://github.com/SumiMakito/AwesomeQRCode-Kotlin |
Python | https://github.com/sylnsfar/qrcode |
Contributors
This project exists thanks to all the people who contribute. [Contribute]
PS: We use QRCodeSwift to generate QR code on watchOS, thanks to ApolloZhu.
Donations
If you think this project has brought you help, you can buy me a cup of coffee. If you like this project and are willing to provide further support for it’s development, you can choose to become Backer
or Sponsor
in Open Collective.
Backers
Thank you to all our backers! 🙏 [Become a backer]
Efr Codec
Sponsors
Support this project by becoming a sponsor. Your logo will show up here with a link to your website. [Become a sponsor]
Thanks for your support, 🙏
Thanks
- Thanks for the help from JetBrains’s Open Source Support Program.
Apps using EFQRCode
Other
Part of the pictures in the demo project and guide come from the internet. If there is any infringement of your legitimate rights and interests, please contact us to delete.
Contact
Email: eyrefree@eyrefree.org
License
EFQRCode is available under the MIT license. See the LICENSE file for more info.
NOTE: Want to explore EFQRCode interactively? Get our user guide as Swift Playground here.
1. Recognition
There are two equivalent ways:
or
Because of the possibility that more than one QR code exist in the same image, the return value is a [String]
. If the returned array is empty, we could not recognize/didn’t find any QR code in the image.
2. Generation
Again, there are two equivalent ways of doing this:
or
The return value is of type CGImage?
. If it is nil
, something went wrong during generation.
Parameters Explained
content: String?
Content is a required parameter, with its capacity limited at 1273 characters. The density of the QR-lattice increases with the increases of the content length. For example:
10 characters | 250 characters |
---|
mode: EFQRCodeMode
Mode of QR Code is defined as EFQRCodeMode
:
nil | grayscale | binarization |
---|
binarization threshold:
mode nil | 0.3 | 0.5 | 0.8 |
---|
inputCorrectionLevel: EFInputCorrectionLevel
Percent of tolerance (which we default to H defined below) has 4 different levels: L 7% / M 15% / Q 25% / H 30%.
Comparison of different input correction levels (generating for the same content):
L | M | Q | H |
---|
size: EFIntSize
NOTE: will be ignored if magnification is not nil
Length and height of the generated QR code, defaults to 256 by 256. EFIntSize
is just like CGSize
, but width
and height
are Int
instead of CGFloat
.
234 * 234 | 312 * 234 |
---|
magnification: EFIntSize?
Magnification is defined as the ratio of actual size to the smallest possible size, and defaults to nil
. Free mac os x server download.
Directly setting the size
parameter results in low resolution QR code images, so setting the magnification
is recommended instead. If you already have a desired size in mind, we have two helpers methods at your disposal to calculate the magnification that results in the closet dimension: EFQRCodeGenerator.maxMagnification(lessThanOrEqualTo:)
and EFQRCodeGenerator.minMagnification(greaterThanOrEqualTo:)
.
size 300 | magnification 9 |
---|
backgroundColor: CIColor/CGColor
Background color, defaults to white.
foregroundColor: CIColor/CGColor
Foreground color (for code points), defaults to black.
Foreground color set to red | Background color set to gray |
---|
icon: CGImage?
Icon image in the center of QR code image, defaults to nil
.
iconSize: CGFloat?
Size of icon image, defaults to 20% of QR code size if nil
.
Default 20% size | Set to 64 |
---|
watermark: CGImage?
Background watermark image, defaults to nil
.
watermarkMode: EFWatermarkMode
Position of watermark in the QR code, defaults to EFWatermarkMode.scaleAspectFill
. Think of the generated QR code like UIImageView
and EFWatermarkMode
as UIView.ContentMode
.
isWatermarkOpaque: Bool
Treat watermark image as opaque, defaults to false
(use transparency).
Efa Code In Mplus
false | true |
---|
pointOffset: CGFloat
WARNING: Generated QR code might be hard to recognize with this parameter.
Foreground point offset, defaults to 0.
0 | 0.5 |
---|
pointShape: EFPointShape
Shape of foreground code points, defaults to EFPointShape.square
.
square | circle | diamond |
---|
3. Generation from GIF
First you should get the complete Data
of a GIF file
NOTE: You shall not get Data
from UIImage
as it only provides the first frame.
Then you can create GIF QRCode with EFQRCode.generateGIF
:
Parameter | Description |
---|---|
generator | REQUIRED, an EFQRCodeGenerator instance with other settings |
data | REQUIRED, encoded input GIF |
delay | Output QRCode GIF delay, emitted means no change |
loopCount | Times looped in GIF, emitted means no change |
The generator
here is an instance of EFQRCodeGenerator
, as demonstrated above, for configuring other parameters for individual frames in GIF. You can checkout the demo projects for more information.
The result (seizure WARNING) will be something like this:
Now you can get the complete data of output QRCode GIF, next we can save it to local path / system photo library / upload to server or some other things you want to do;
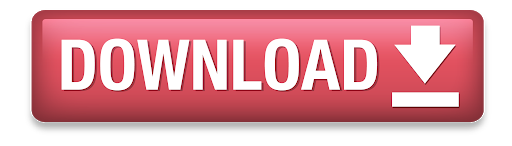